Current time in IST and BST. Time zone converters for IST and BST. Countries in IST and BST. Similar conversions between your chosen time zones. Converting UTC to BST This time zone converter lets you visually and very quickly convert UTC to BST and vice-versa. Simply mouse over the colored hour-tiles and glance at the hours selected by the column. UTC stands for Universal Time. British Summer Time is 1 hour ahead from the UTC universal time. UTC to BST Time Conversion Table UTC to BST in 12-hour (AM/PM) time format. UTC to BST in 24-hour time format.
US, Canada, Mexico Time ZonesEclipse software for java. Atlantic Daylight Time (ADT) • Eastern Daylight Time (EDT) • Central Daylight Time (CDT) • Mountain Daylight Time (MDT) • Pacific Daylight Time (PDT) • Alaska Daylight Time (AKDT) • Hawaii Time • Arizona • Saskatoon • New York • Toronto • Mexico City • San Francisco • Chicago • Houston • Miami • Phoenix • Halifax • Denver • Monterrey • Chihuahua
Europe Time Zones
Greenwich Mean Time (GMT) • British Summer Time (BST) • Western European Summer Time (WEST) • Central European Summer Time (CEST) • Eastern European Summer Time (EEST) • London • Paris • Berlin • Athens • Warsaw • Kiev • Belarus • Moscow • Madrid • Stockholm • Amsterdam • Istanbul
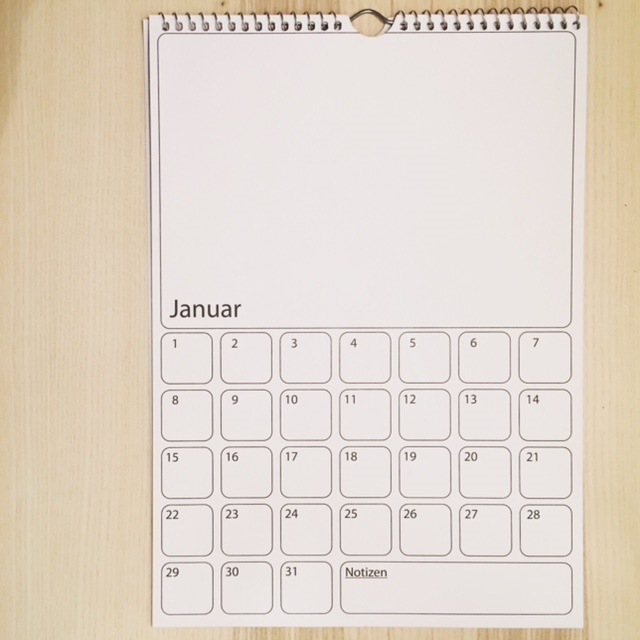
Europe Time Zones
Greenwich Mean Time (GMT) • British Summer Time (BST) • Western European Summer Time (WEST) • Central European Summer Time (CEST) • Eastern European Summer Time (EEST) • London • Paris • Berlin • Athens • Warsaw • Kiev • Belarus • Moscow • Madrid • Stockholm • Amsterdam • Istanbul
Australia, New Zealand Time Zones
AEST • ACST • AWST •New Zealand Time (NZT) • Queensland • Adelaide • Brisbane • Canberra • Melbourne • Perth • Sydney • Auckland • Fiji • Solomon Islands • Papua New Guinea
Asia Time Zones
India • Pakistan • China • UAE • Japan • Korea • Philippines • Thailand • Hong Kong • Taiwan • Malaysia • Singapore • Jakarta • Bangladesh • Sri Lanka • Nepal • Kuwait • Saudi Arabia • Viet Nam • Oman • Israel • Jordan • Beijing • Bangalore • Kuala Lumpur • Manila • Tokyo • Seoul • Karachi • Dubai
Africa Time Zones
West Africa Time (WAT) • Central Africa Time (CAT) • East Africa Time (EAT) • Egypt • Nigeria • Kenya • Ghana • Morocco • Tanzania • Ethiopia • Uganda • South Africa • Cairo • Algiers • Casablanca • Accra • Lagos • Cape Town • Nairobi
South America Time Zones
Brazil • Argentina • Chile • Peru • Ecuador • Colombia • Venezuela • Panama • Puerto Rico • São Paulo • Manaus • Rio de Janeiro • Buenos Aires • Santiago • Lima • Quito • Bogota • Caracas
Russia Time Zones
Moscow • Novosibirsk • Yekaterinburg • Omsk • St Petersburg • Kazan • Irkutsk • Chita • Vladivostok • Sochi • Almaty • Kyrgyzstan • Uzbekistan • Tajikistan
Given a BST (Binary Search Tree) that may be unbalanced, convert it into a balanced BST that has minimum possible height.
Examples :
A Simple Solution is to traverse nodes in Inorder and one by one insert into a self-balancing BST like AVL tree. Time complexity of this solution is O(n Log n) and this solution doesn't guarantee Netbeans eclipse.
An Efficient Solution can construct balanced BST in O(n) time with minimum possible height. Below are steps.
- Traverse given BST in inorder and store result in an array. This step takes O(n) time. Note that this array would be sorted as inorder traversal of BST always produces sorted sequence.
- Build a balanced BST from the above created sorted array using the recursive approach discussed here. This step also takes O(n) time as we traverse every element exactly once and processing an element takes O(1) time.
Below is the implementation of above steps.
C++
// C++ program to convert a left unbalanced BST to
// a balanced BST
#include
// Store nodes in Inorder (which is sorted
// order for BST)
storeBSTNodes(root->left, nodes);
nodes.push_back(root);
storeBSTNodes(root->right, nodes);
}
/* Recursive function to construct binary tree */
Node* buildTreeUtil(vector &nodes, int start,
int end)
{
// base case
if (start > end)
return NULL;
Ust To Bst Time
/* Get the middle element and make it root */
int mid = (start + end)/2;
Node *root = nodes[mid];
/* Using index in Inorder traversal, construct
left and right subtress */
root->left = buildTreeUtil(nodes, start, mid-1);
root->right = buildTreeUtil(nodes, mid+1, end);
return root;
}
// This functions converts an unbalanced BST to
// a balanced BST
Node* buildTree(Node* root)
{
// Store nodes of given BST in sorted order
vector
// Constucts BST from nodes[]
int n = nodes.size();
return buildTreeUtil(nodes, 0, n-1);
}
// Utility function to create a new node
Node* newNode(int data)
{
Node* node = new Node;
node->data = data;
node->left = node->right = NULL;
return (node);
}
/* Function to do preorder traversal of tree */
void preOrder(Node* node)
{
if (node NULL)
return;
printf('%d ', node->data);
preOrder(node->left);
preOrder(node->right);
}
// Driver program
int main()
{
/* Constructed skewed binary tree is
10
/
8
/
7
/
6
/
5 */
Node* root = newNode(10);
root->left = newNode(8);
root->left->left = newNode(7);
root->left->left->left = newNode(6);
root->left->left->left->left = newNode(5);
root = buildTree(root);
printf('Preorder traversal of balanced '
'BST is :');
preOrder(root);
return 0;
}
Java
Ust To Bst Transfer
// Java program to convert a left unbalanced BST to a balanced BST import java.util.*; /* A binary tree node has data, pointer to left child class Node int data; { left = right = null ; } class BinaryTree Node root; /* This function traverse the skewed binary tree and stores its nodes pointers in vector nodes[] */ void storeBSTNodes(Node root, Vector nodes) // Base case return ; // Store nodes in Inorder (which is sorted storeBSTNodes(root.left, nodes); storeBSTNodes(root.right, nodes); /* Recursive function to construct binary tree */ Node buildTreeUtil(Vector nodes, int start, { if (start > end) /* Get the middle element and make it root */ Node node = nodes.get(mid); /* Using index in Inorder traversal, construct node.left = buildTreeUtil(nodes, start, mid - 1 ); node.right = buildTreeUtil(nodes, mid + 1 , end); return node; // This functions converts an unbalanced BST to Node buildTree(Node root) // Store nodes of given BST in sorted order storeBSTNodes(root, nodes); // Constucts BST from nodes[] return buildTreeUtil(nodes, 0 , n - 1 ); /* Function to do preorder traversal of tree */ { return ; preOrder(node.left); } // Driver program to test the above functions { 10 8 7 6 5 */ tree.root = new Node( 10 ); tree.root.left.left = new Node( 7 ); tree.root.left.left.left.left = new Node( 5 ); tree.root = tree.buildTree(tree.root); System.out.println( 'Preorder traversal of balanced BST is :' ); } // This code has been contributed by Mayank Jaiswal(mayank_24) |
C#
using System.Collections.Generic; // C# program to convert a left unbalanced BST to a balanced BST /* A binary tree node has data, pointer to left child public class Node public int data; { left = right = null ; } public class BinaryTree public Node root; /* This function traverse the skewed binary tree and stores its nodes pointers in vector nodes[] */ public virtual void storeBSTNodes(Node root, List nodes) // Base case { } // Store nodes in Inorder (which is sorted storeBSTNodes(root.left, nodes); storeBSTNodes(root.right, nodes); /* Recursive function to construct binary tree */ public virtual Node buildTreeUtil(List nodes, int start, int end) // base case { } /* Get the middle element and make it root */ Node node = nodes[mid]; /* Using index in Inorder traversal, construct node.left = buildTreeUtil(nodes, start, mid - 1); node.right = buildTreeUtil(nodes, mid + 1, end); return node; // This functions converts an unbalanced BST to public virtual Node buildTree(Node root) // Store nodes of given BST in sorted order storeBSTNodes(root, nodes); // Constucts BST from nodes[] return buildTreeUtil(nodes, 0, n - 1); /* Function to do preorder traversal of tree */ { { } preOrder(node.left); } // Driver program to test the above functions { 10 8 7 6 5 */ tree.root = new Node(10); tree.root.left.left = new Node(7); tree.root.left.left.left.left = new Node(5); tree.root = tree.buildTree(tree.root); Console.WriteLine( 'Preorder traversal of balanced BST is :' ); } |
Output :
Ust Bstm
Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above